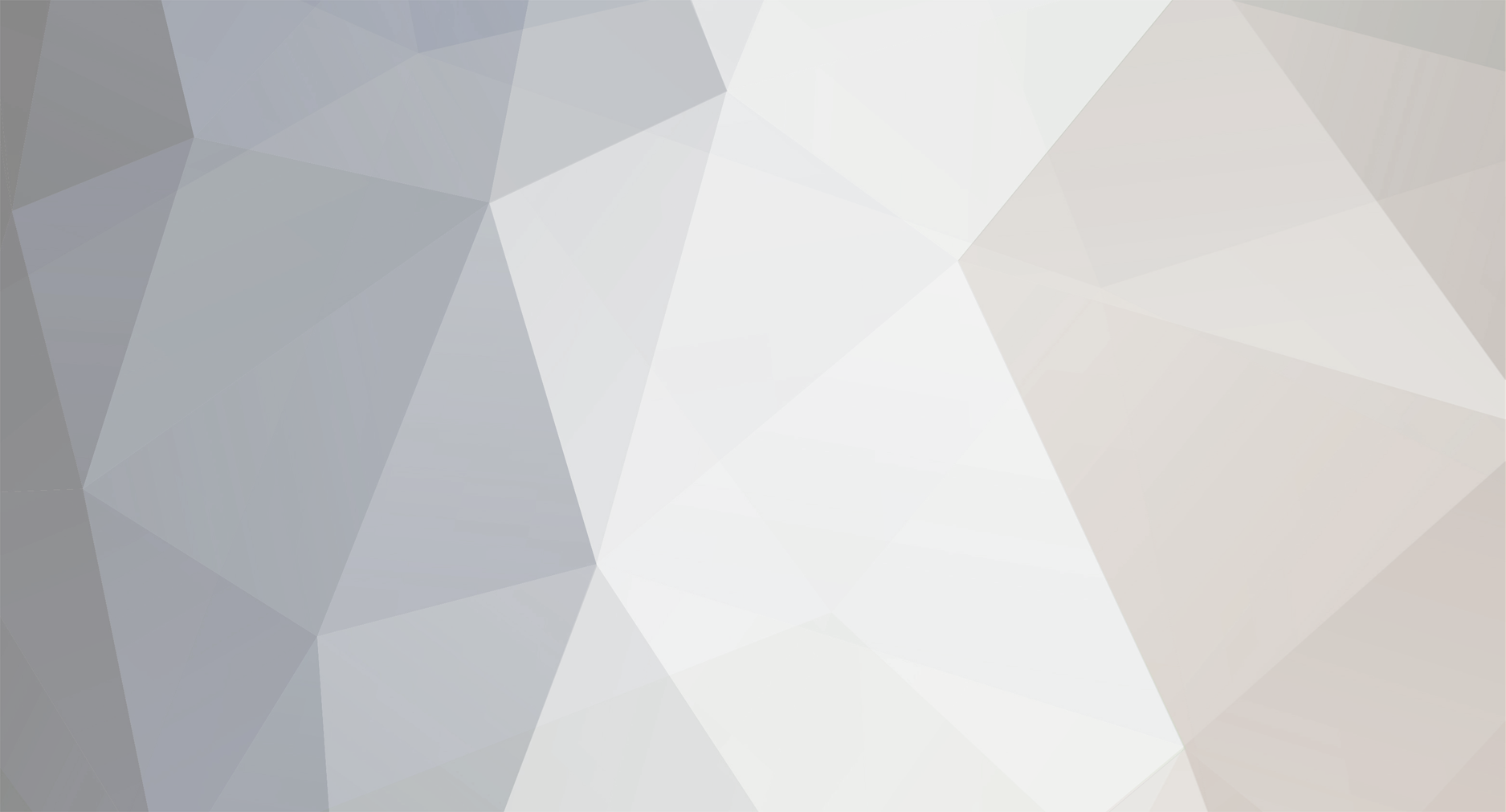
Zaffin_D
-
Posts
374 -
Joined
-
Days Won
1
Content Type
Profiles
Forums
Downloads
Store
eMastercam Wiki
Blogs
Gallery
Events
Posts posted by Zaffin_D
-
-
Are you using the Siemens 3x post (older, no longer supported by CNC) or the Siemens 3x_4x post (currently supported)?
Also not every Siemens control is supported, what control are you using?
-
1
-
-
1 hour ago, GoetzInd said:
Unfortunately, still hanging up on axis sub ops in 2020.
Mike
Is a rapid move being wrapped? If so it's a post issue. @Chally72 can get it to the right team I'm sure.
-
1
-
-
7 hours ago, Norbert Dalhoff said:
Hello again,
trying to access Mastercam Plane which is not one of the reserved, i got Always errors. Don't know why.
This code works:
const Plane *arcPlane = Planes.GetPlanePtrByID(1); //get-Plane 1==TOP swprintf(sztext, L"Name of Plane %s\n", arcPlane->GetName()); //tell me name, WORKS and gives TOP
and this didn't work with existing plane No. 22. plane 22 is in planemanager visible and ok but
this code CRASHES:
const Plane *arcPlane = Planes.GetPlanePtrByID(22); //get Plane No. 22 swprintf(sztext, L"Name of Plane %s\n", arcPlane->GetName()); //-----------------------CRASH
Do you know why?
Thanks in advance for any help or hint.
What version of Mastercam are you using? In Mastercam 2020 the GetPlanePtrByID() function works as expected in my testing.
Can you share a file?
C++,
Why are you using a char array? And why so big? 5000 bytes of continuous memory seems excessive.
-
Typically I create a global alias for the variable and format it, but you can also use the new address function nwadrs( ); just remember to set the format back after output.
-
1
-
-
I suppose you could read/write the value to/from a text file, something like the below;
return : 0 my_setting : 0 fs2 4 1 0 1 0 fmt "" 4 my_setting fbuf 1 1 1 1 0 fq 1 my_setting "Enter a number" p_load_question_defaults spathaux$ = smc_user_dir$ snameaux$ = "questionDefaults" sextaux$ = ".txt" sbufname1$ = spathaux$ + snameaux$ + sextaux$ if not(fexist(sbufname1$)), [ auxprg$ = 1 subout$ = 2 newaux$ *my_setting, e$ return = fclose(sbufname1$) subout$ = 0 ] else, [ my_setting = rbuf(1,1) ] p_save_question_defaults my_setting = wbuf(1,1) pheader$ p_load_question_defaults "Default value read from file :", *my_setting, e$ q1 "Value entered in prompt :", *my_setting, e$ p_save_question_defaults
-
I had some time today so I dug into this.
I started by drawing a 1" diameter arc in the Top view. I then copy-rotated the arc about the Y-axis giving me two arcs shown below.
I wrote a quick hook to query the arcs, results below.
There are a couple ways to work around this. The best thing would be to create a CLR project and call the C-Hook SDK's get_view_matrix function as shown in the .gif above, but you cold also fake it.
You can fake it without using the C-Hook SDK, but the calculated matrix probably won't match the matrix from get_view_matrix; see the CreateViewFromArc(ArcGeometry arc) method below.
namespace ArcMatrixExampleManaged { using System.Linq; using Mastercam.IO; using Mastercam.Database; using Mastercam.Database.Types; using Mastercam.Curves; using Mastercam.App; using Mastercam.App.Types; using Mastercam.Support; using Mastercam.Math; using ArcServiceNative; public class Main : NetHook3App { bool CreateViewFromArc(ArcGeometry arc) { arc.Data.StartAngleDegrees = 0; arc.Data.EndAngleDegrees = 90; var vectorOne = VectorManager.Normalize(arc.EndPoint1); var vectorTwo = VectorManager.Normalize(arc.EndPoint2); var vectorThree = VectorManager.Normalize(Point3D.Cross(vectorOne, vectorTwo)); var arcView = new MCView() { ViewName = $"Arc View[{arc.TimeStamp}]", ViewOrigin = arc.Data.CenterPoint, ViewMatrix = new Matrix3D(vectorOne, vectorTwo, vectorThree) }; return arcView.Commit(); } public override MCamReturn Run(int param) { using (var arcService = new ArcService()) { var arcMask = new GeometryMask(false) { Arcs = true }; var selectedArc = (ArcGeometry)SelectionManager.AskForGeometry("Select and Arc", arcMask); if (selectedArc != null) { var arcViewMatrix = selectedArc.View.ViewMatrix; var managedViewMatrix = SearchManager.GetViews(selectedArc.ViewNumber).FirstOrDefault(); var nativeViewMatrix = arcService.GetViewMatrix(selectedArc.ViewNumber); DialogManager.OK("View Matrix from ArcGeometry object\n" + $" {arcViewMatrix.Row1.ToString()}\n" + $" {arcViewMatrix.Row2.ToString()}\n" + $" {arcViewMatrix.Row3.ToString()}\n" + $"\n" + $"View Matrix from SearchManager.GetViews\n" + $" {managedViewMatrix?.ViewMatrix.Row1.ToString()}\n" + $" {managedViewMatrix?.ViewMatrix.Row2.ToString()}\n" + $" {managedViewMatrix?.ViewMatrix.Row3.ToString()}\n" + $"\n" + $"View Matrix from native get_view_matrix\n" + $" X{nativeViewMatrix.AxisX.X.ToString("0.0000")} Y{nativeViewMatrix.AxisX.Y.ToString("0.0000")} Z{nativeViewMatrix.AxisX.Z.ToString("0.0000")}\n" + $" X{nativeViewMatrix.AxisY.X.ToString("0.0000")} Y{nativeViewMatrix.AxisY.Y.ToString("0.0000")} Z{nativeViewMatrix.AxisY.Z.ToString("0.0000")}\n" + $" X{nativeViewMatrix.AxisZ.X.ToString("0.0000")} Y{nativeViewMatrix.AxisZ.Y.ToString("0.0000")} Z{nativeViewMatrix.AxisZ.Z.ToString("0.0000")}\n", "Arc View Report"); var result = CreateViewFromArc(selectedArc); } } return MCamReturn.NoErrors; } } }
-
Are you trying to create a view from a rotated arc?
If so you'll find that if the arc's view matrix is incorrect if the arc's view isn't in the database.
I do have a few solutions; let me know if I'm on the right track and all post them.
-
I'd make sure there isn't anything unexpected in your output, maybe open the .nc file with a hex editor?
-
1 hour ago, c++ said:
No, the problem comes when I run one of the functions, then for example move the 3d model and run it again. Strange this is not the case for you.
Ahh I see the issue! The alive bit gets manipulated when moving geometry and when removing a solids history. Checking to make sure the solid is alive seems to solve the issue.
std::vector<DB_LIST_ENT_PTR> GetAllSolids() { int numberOfSolidsSelected = 0; std::vector<DB_LIST_ENT_PTR> dblistptrvector; auto entityPointer = db_start; while (entityPointer != nullptr) { if (entityPointer->eptr->id == SOLID_ID && entityPointer->eptr->sel & ALIVE_BIT) { if (sel_bit_is_off(BLANK_BIT, entityPointer->eptr->sel)) { numberOfSolidsSelected++; dblistptrvector.push_back(entityPointer); } } entityPointer = entityPointer->next; } if (numberOfSolidsSelected) { repaint_graphics(); } return dblistptrvector; }
-
In my half hour of testing both functions return the same values, I've tried blanking and hiding entities, removing them, adding non solids; they return the same vector.
Are you testing the functions outside of your project?
-
On 1/25/2020 at 1:32 PM, c++ said:
Nevermind, anyway I found the source of the problem, back in business.
I looked over the commit and nothing jumps out at me as fixing the problem.
It did raise two questions though;
- Why are you using memset?
- Why are you using NULL and not nullptr?
-
1 hour ago, c++ said:
Please feel free to download it, the function you probably want to look at is -> void dogetgeometry()
I assume you mean std::vector<double>dogetgeometry()? I could not find a void overload for dogetgeometry().
This project is in quite the state and I don't have time to dissect it (again); I'm sure someone else can help you out.
-
36 minutes ago, c++ said:
Oops I edited the gcnew bit in amd the end forgot to drop the const
I don't know exactly where, but the memory for my geometry isn't getting freed, if I program the panel using the macro then move it, it will continue to program in the same spot. I can even "import" the geometrt from one file to another.
Without the entire project and steps to reproduce this is going to be tough to track down.
My gut says this is not a memory issue.
-
7 hours ago, c++ said:
In the case of vectors It seems to be okay, but when it comes to .net lists the data needs to be cleared, even if the list is not initialized with the new keyword.
What makes you think this?
QuoteThe handle declarator (^, pronounced "hat"), modifies the type specifier to mean that the declared object should be automatically deleted when the system determines that the object is no longer accessible.
The garbage collector should manage the memory; what issue are you having?
Also your example has an issue, the below throws an 'E0137 expression must be a modifiable lvalue' error.
const auto chains = Mastercam::Database::ChainManager::ChainAll(true, false, nullptr, Mastercam::Database::Types::ChainDirectionType::Clockwise, level4); chains->Clear; //You declared chains as const, so you can't reassign it. chains = gcnew array< Mastercam::Database::Chain^ >(0);
-
4 hours ago, Grimes said:
ive actually got my post for the mazak finished, didnt take much just the feed thing is killing me.
Can you upload the post? I'm not an expert but I think I can point you in the right direction.
-
1
-
-
3 hours ago, Kalibre said:
ptap$ #Canned Tap Cycle pdrlcommonb result = newfs(17, feed) # this reformat statement could be messing it up if met_tool$, [ if toolismetric, pitch = n_tap_thds$ else, pitch = (1/n_tap_thds$) * 25.4 ] else, [ if toolismetric, pitch = n_tap_thds$ * (1/25.4) else, pitch = 1/n_tap_thds$ ] pitch = pitch * speed # this section is def forcing ipm, comment this line out pbld, n$, sg94, e$ # since you don't use G94/95, comment this line out pcan1, pbld, n$, *sgdrlref, *sgdrill, pxout, pyout, pfzout, pcout, prdrlout, *pitch, !feed, strcantext, e$ pcom_movea
If your ptap$ section looks like this, try making the commented modifications and run it again. I'm guessing all the post warnings you got were from the global calculation running with a null spindle speed. The !feed can probably go tho.
That logic isn't the best (I'm aware it came from a generic); In Mastercam 2019 and beyond toolismetric will never be true. Beside that, using the tap_pitch$ variable will half the amount of code in that postblock.
OP, have you contacted your reseller? If you need this in a hurry I think that would be your best bet.
-
Are they C-hooks (written in C++) or NET-Hooks (written in C#/VB.net)?
If it's a C-hook are you building the release version?
No errors is suspicious; if you run an invalid .dll Mastercam should tell you.
-
20 minutes ago, content creator said:
If the contents are being stored on the heap, what I have read about the heap, slower performance, memory fragmentation, It seems like vectors are probably good for new programmers to manage memory, but we will need to evolve. I wonder what the implications are of storing a vector of c-arrays a specific size, maybe map would be better.
I'm not an expert, but this likely isn't something you need to worry about.
A (very) wise women once said "If you don't know what type of container you need, use a vector".
If you take advantage of lambdas and iterators you can likely measure the performance between any of the STL containers just by changing the type.
-
42 minutes ago, content creator said:
This is where I read that the memory is actually allocated on the heap.
The vector is on the stack and the contents are on the heap. You did not allocate the heap memory so you do not need to free it. When the vector goes out of scope the memory will be freed. I don't see a reason to worry about using a smart pointer in the above example.
I'm not sure what your trying to do in the above snippet, or what the issue is.
When you look at the Mastercam SDK remember that Mastercam is 35 years old. Most of the newer functions seem to use the STL stuff. Alot of MFC in there too.
-
36 minutes ago, content creator said:
Thanks for the info.. I've been reading a lot about this,
right now I write everything in c++/cli project using various c# and c++ libraries.
I store the bulk of my data in vectors declared like
int get_size() { std::vector<int> vector_of_ints; int randomnumber = 1000; for(auto i = 0; i < randomnumber; i++;) { vector_of_ints.push_back(i); } //do something with the vector return i; }
I guess that technically stores the data on the heap then it gets deallocated when the function goes out of scope, If I am understanding correctly would a vector be a good place to use a smart pointer, or would you recommend an array?
Nope, that vector is on the stack; it's lifetime is from declaration till it goes out of scope. In the above example I see no reason to change anything.
I would not use a C-style array; I prefer the containers from the standard library.
-
On 1/15/2020 at 6:05 PM, content creator said:
Would it be possible for you to provide me an example of what you meant by this? I would like to improve.
Maybe? Cpp isn't my jam, but I'll do my best.
Consider the below code...
#include <iostream> #include <string> #include <memory> class Point { public: std::string Name; double X; double Y; Point(); Point(std::string pointName, double xPosition, double yPosition); ~Point(); }; Point::Point() { Name = "Default Name"; X = 0.0; Y = 0.0; } Point::Point(std::string pointName, double xPosition, double yPosition) : Name(pointName), X(xPosition), Y(yPosition) { } Point::~Point() { std::cout << "Destructing " << Name << std::endl; } int main() { /*Curly braces define scope; this will allow us to see what happends when the below objects go out of scope. */ { // A point object created on the stack. Point pointOne{ "Point One", 1.0, 1.0 }; // A raw pointer created on the heap. auto pointTwo = new Point("Point Two", 2.0, 2.0); // A smart pointer. auto pointThree = std::make_unique<Point>("Point Three", 3.0, 3.0); } } // Output: // Destructing Point Three // Destructing Point One
Did you notice that the destructor for pointTwo wasn't called? Because it was created with the 'new' keyword, it's our responsibility to clean it up before it goes out of scope.
This issue can largely be avoided by using smart pointers; pointThree is an example of a smart pointer.
I suggest you check out google; there are many good videos on this subject.
-
33 minutes ago, Joe777 said:
If your have searched for "M88" in the Post and can't find it. Machine Definition is a place to look.
I'll elaborate; it is possible to read the strings in from that dialog, I've just never seen it done. Typically the coolant m-code output is handled by a string select table inside the post.
I asked if you tested modifying the enable and disable labels because I'm curious if they are wired up.
-
-
tabison is boolean; if...else would be a bit more traditional.
Thanks for the offer, but I'm good. Too much learning wise on my plate already.
Siemens 3x mill post
in Post Processor Development Forum
Posted
You should head over to Mastercam's tech exchange and download the Siemens 3x_4x mill post; the post you have is the older unsupported version.